🔍 Search
📥 Subscribe
Parameter Tampering Attack
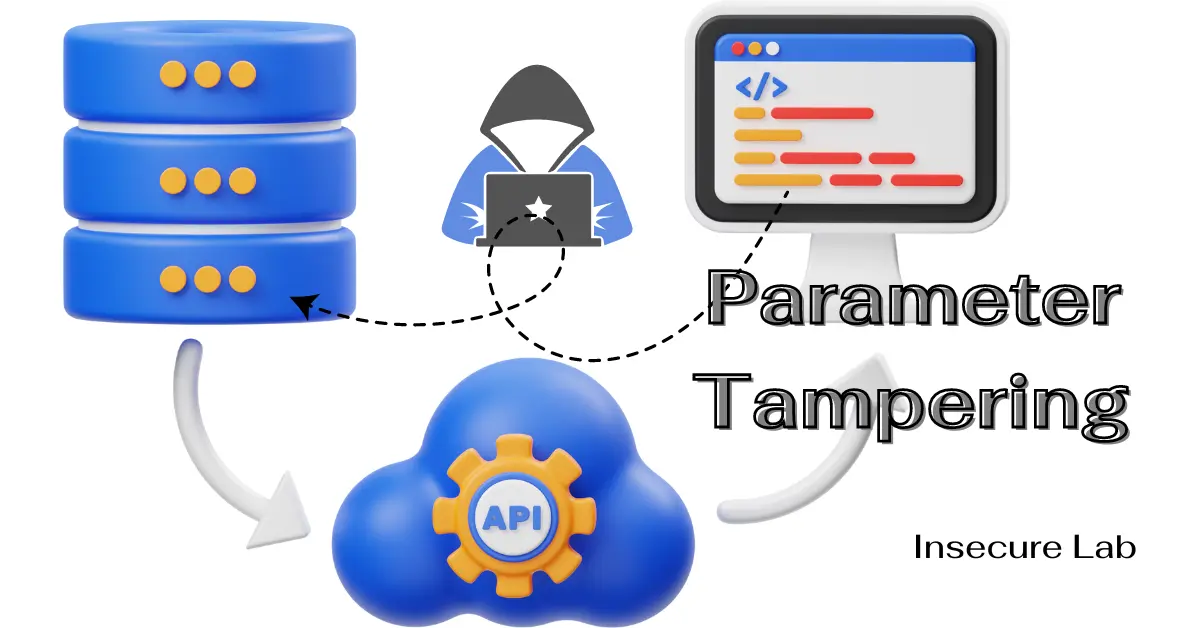
Table of Contents
This guide explores the concept of parameter tampering, types of attacks, examples of API and form tampering, and prevention techniques in cyber security.
What is Parameter Tampering?
Parameter tampering is a form of web attack where an attacker modifies parameters exchanged between a client and a server in order to exploit the application. This malicious activity can lead to various security issues such as unauthorized access, privilege escalation, system disruption, data breach, and financial fraud.
Understanding Parameters
Web applications rely on parameters to control their behavior and process user input. These parameters can be found in various locations, including:
-
URL Query Strings: Appended to the URL after a question mark '
?
', they hold key-value pairs, like 'product_id=123
'. - HTTP Headers: Additional information sent with requests, such as authentication tokens.
- Cookies: Small pieces of data stored on the user's device to maintain session state.
- Form Fields: User-submitted data through web forms.
Types of Parameter Tampering Attacks
1. URL Tampering
By changing the URL parameters, attackers can attempt to bypass authentication, access restricted areas, or exploit vulnerabilities within the web application.
2. Form Tampering
Form tampering happens when an attacker modifies the elements of a web form before submission. This could include changing the value of a hidden field that controls the price of a product or the user ID in a profile update form.
3. API Tampering
API tampering involves manipulating the API requests sent from the client to the server. Attackers can alter parameters in the REST API call to access or modify unauthorized information.
4. HTTP Header Tampering
Modifying headers can affect the behavior of the application, leading to attacks such as spoofing the referrer header to gain unauthorized access.
5. Cookie Tampering
Cookies often store session data, including user preferences or authentication tokens. Tampering with cookie values can lead to session hijacking, where an attacker assumes the identity of a legitimate user.
Examples using Python
• Form Parameter Tampering
Consider an online shopping website where the price of a product is determined on the client side through a hidden form field. An attacker could tamper with this form to modify the price before it is submitted to the server.
Vulnerable HTML Form:
<form action="/purchase" method="POST"> <input type="hidden" name="productID" value="1234"> <input type="hidden" name="price" value="99.99"> <input type="submit" value="Buy Now"> </form>
Python Code to Simulate Form Tampering:
import requests # URL of the web application's purchase endpoint url = 'http://example.com/purchase' # Data with tampered price data = {'productID': '1234', 'price': '0.01'} # Sending tampered data as if submitted through the form response = requests.post(url, data=data) print(response.text)
• API Parameter Tampering
Consider a banking application's API for transferring funds. An attacker can increase the transaction amount by modifying the "amount" parameter in the REST API request to transfer a larger amount than intended.
Python Code to Simulate API Tampering:
import requests # Replace with actual API endpoint and authentication details api_url = 'https://vulnerable_bank.com/api/transfer' headers = {'Authorization': 'your_api_key'} # Malicious modification of transfer amount malicious_data = {'from': '123456', 'to': '987654', 'amount': 10000} # Change amount to a larger value response = requests.post(api_url, headers=headers, json=malicious_data) if response.status_code == 200: print("Attack may be successful. Check transaction logs.") else: print("Attack failed. Server might have input validation.")
Prevention and Mitigation
- Implement robust validation on the server-side to ensure parameters meet expected format, length, and range.
- Sanitize all user input to remove potentially malicious code before processing.
- Use URL encoding for parameters to prevent special characters from being misinterpreted.
- Implement strong authorization mechanisms to restrict access based on user roles and permissions.
- Conduct penetration testing and security audits to identify and address parameter tampering vulnerabilities.
- Enforce authentication, authorization, and rate limiting for API interactions.
- Use HTTPS to encrypt data transmission and prevent eavesdropping.
- Implement secure session management with strong tokens, regular expiration, and invalidation on logout to prevent session hijacking.
- Use parameterized queries and prepared statements to prevent SQL injection attacks.